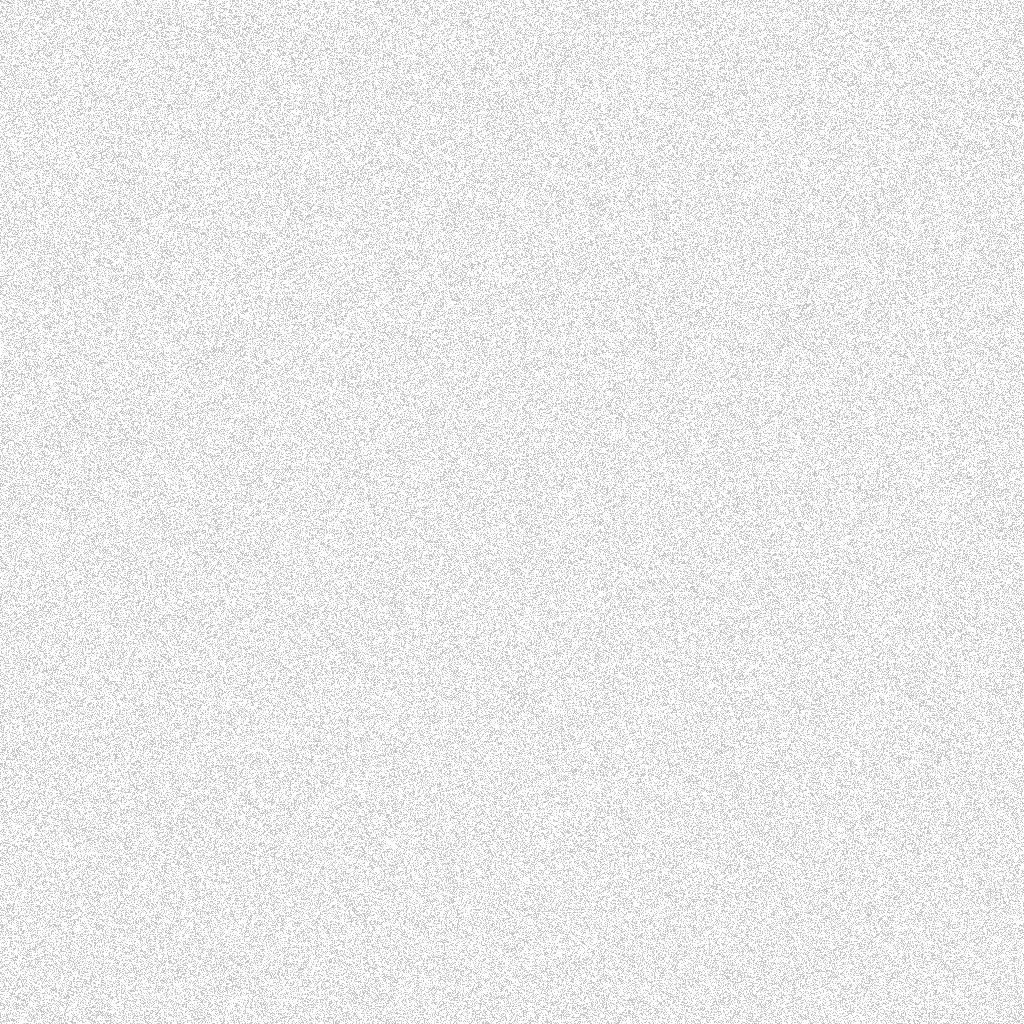

Focus/Keyboard Nav Utilities @ Smartsheet
Building tools for accessible focus/keyboard nav management across Smartsheet UIs
โ๐ฝ About Smartsheet
Smartsheet is an industry-leading enterprise work management platform, boasting millions of passionate users!
The same project data can be dynamically viewed in various different ways (card/boards, timelines/gantt charts, and tables/grids). Content/asset management, workflows, mini-apps, dashboards, and more make for a robust CWM ecosystem capable of servicing large enterprise customers and small nimble customers alike.
โ ๏ธ Keyboard Navigation & Accessibility
Programmatic focus/keyboard nav is a staple a11y concern across components in a design system. In complex components, baking in accessible keyboard navigation with sensical screen reader interactions out of the box can be a huge value-add for:
- Consumers of the system, who get to save development time & effort building those things in themselves
- End users, who get to benefit from good usability and interface accessibility
Many of our components at Smartsheet ship keyboard nav capabilities out of the box, such as our Toolbar
and Menu
components, which allow for arrow key navigation between items and more. In recent work, I was brushing up our Menu's focus/keyboard nav code which led me to create a reusable utility to help handle programmatic keyboard nav and focus - the topic of this post ๐ค
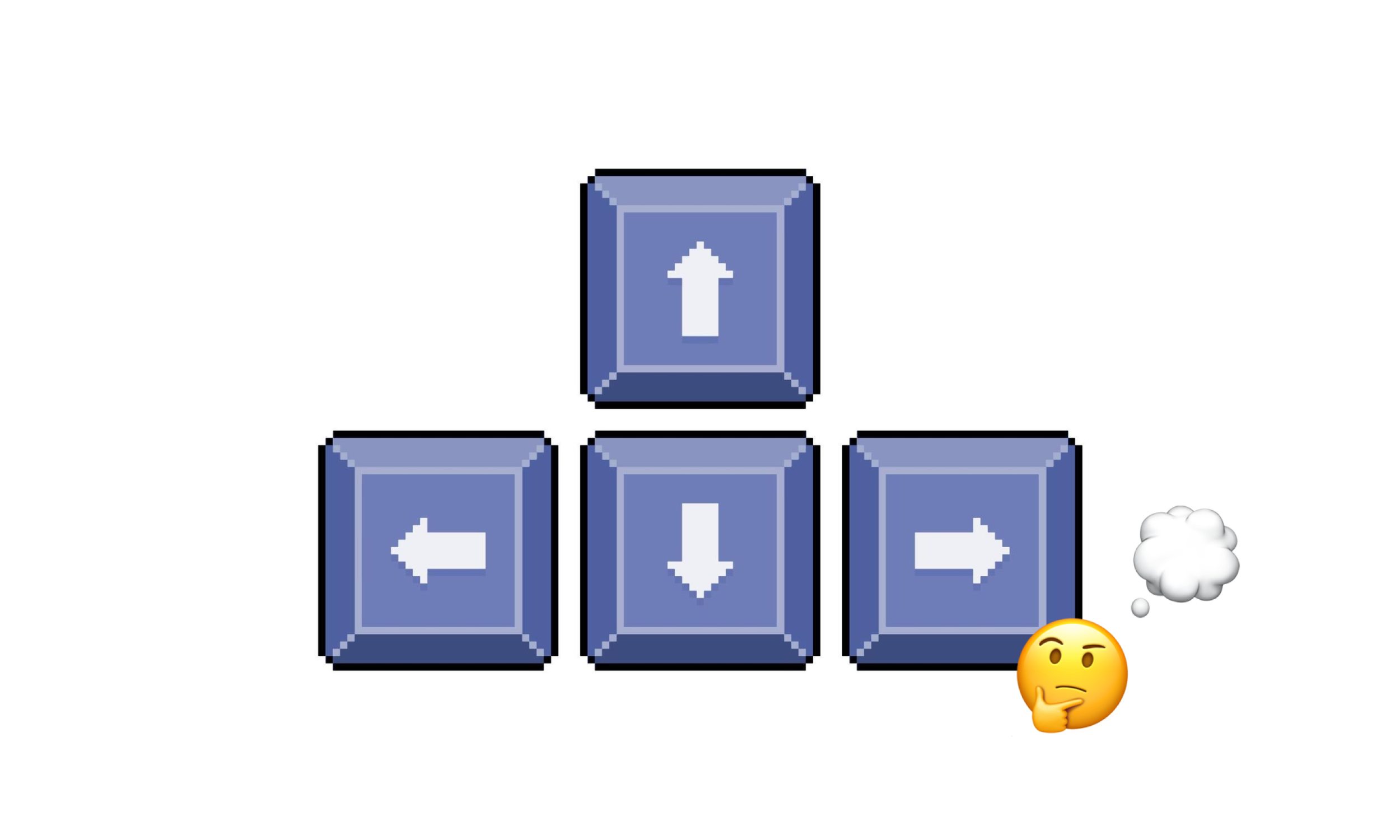
How does programmatic keyboard navigation work?
Programmatic focus management is a very browser-API-centric effort. Generally, if you want to make components easily navigable via keyboard (such as arrow keys in a listbox, etc.) you have to implement a series of keyPress events that traverse DOM nodes in certain ways. In a react component, that might look something like this ๐๐ฝ
//a very crude, oversimplified example :)
const MyComponent = () => {
const items = [1, 2, 3];
const handleKeyDown = (event) => {
if (event.key === "ArrowUp") {
console.log("UP");
// Perform some action
} else if (event.key === "ArrowDown") {
console.log("DOWN");
// Perform some action
} else {
...
}
};
return (
<ul>
{items.map((item) => (
<li onKeydown={handleKeyDown}>{item}</li>
))}
</ul>
);
};
Here, MyComponent
is a container with some items, and those items are sensitive to keypress events, and they perform some sort of logic depending on what the user pressed. But what might that logic look like in more detail? It is the secret sauce here, after all.
//a bit more specific example of keypress handlers for keyboard nav/focus management
//this is just an example demo'ing that SOMEHOW, you've got the active element stored.
const activeElement = document.activeElement;
const handleKeyDown = (event) => {
if (event.key === "ArrowUp") {
//get the prev sibling element
const prevElement = activeElement?.previousElementSibling;
if(prevElement) {
//if it exists, unfocus the current element
//and focus the prev one
activeElement.tabIndex = -1;
prevElement.tabIndex = 0;
prevElement.focus();
}
} else if (event.key === "ArrowDown") {
//get the next sibling element
const nextElement = activeElement?.nextElementSibling;
if(nextElement) {
//if it exists, unfocus the current element
//and focus the next one
activeElement.tabIndex = -1;
nextElement.tabIndex = 0;
nextElement.focus();
}
} else {
...
}
};
Two important things to focus on here (pun very very intended ๐):
- We are updating the tabIndex as we go
- We are manually focusing as we go
The focus() calls are pretty straight forward - move the focus to the next element in question.
The updating of the tabIndex moves things in and out of the page's focus order. If your component contains a bunch of items that take a while to traverse, it can be nice to make sure only one item at a time is a focusable tab stop. The user can use arrow keys to navigate and they can use the tab key to leave that component altogether to traverse to the next interactable element on the page. And even cooler - if they come back to it, it will go right to where they left off because the last item they traversed to is the tab stop for that component (assuming no rerendering, etc.). Neat!
So what's the problem? ๐คจ
Glad you asked. So imagine a real, more complex component than our super simple example above. Imagine various keypresses we may want to account for, etc. Now imagine as well that same code needed across MANY components that need these interaction capabilities. And finally, imagine how many consumers are taking design system components and stitching together product UIs that also need this exact same functionality. Suddenly, we've got an issue of scale and a violation of DRY principles (Don't Repeat Yourself) within our entire frontend infrastructure.
When encountering this in our design system code at Smartsheet recently, I decided to abstract some of this high-repitition code into a reusable utility function, for us as well as our consumers.
focusNewElement() ๐ ๏ธ
This new function is super simple, and really flexible in how it can be used. See for yourself, then let's break it down:
/**
* A utility function that handles programmatic focusing from one element (`currentElement`)
* to another element (`nextElement`). It handles .focus() as well as tabIndex changing.
*
* @param currentElement the current element you're focused on. If the focus is being called
* to an element for the first time and there is no current focused element,
* set this to `null`.
* @param nextElement the next element you want to shift focus to.
* @param tabIndices optional set of 2 numbers which define what the tabIndices will be after
* this focus event takes place. The first number is the new tabIndex for the currentElement
* and the second is the new tabIndex for the nextElement.
*/
export const focusNewElement = (
currentElement: HTMLElement | null,
nextElement: HTMLElement,
tabIndices?: [number?, number?]
) => {
//if a current element is passed in...
if (currentElement) {
//... set the currentElement tabIndex to either the custom tabIndex or -1
currentElement.tabIndex = tabIndices?.[0] !== undefined ? tabIndices[0] : -1;
}
// set the nextElement tabIndex to either the custom tabIndex or 0
nextElement.tabIndex = tabIndices?.[1] !== undefined ? tabIndices[1] : 0;
nextElement.focus();
};
Prop API ๐จ
The API for this function contains just a few args:
currentElement
- the current focused/nav'd elementnextElement
- where you want to focus/nav to nexttabIndices
- the new tabIndex for each element after this new nav/focus action takes place
The logic ๐ก
The function isn't too crazy! If you pass it a currentElement
, it'll do some tabIndex logic on that element before we move focus elsewhere. In either case it moves on, and makes the next element interactable, then focuses to it.
Sometimes though, you may not want to move focus to a new element while setting the tabIndex to -1 & 0 respectively as you traverse. That default behavior here is super helpful in components that contain things like listboxes
, filetree
, menu
, etc. but this function can be used for any other normal focus/keyboard nav event thanks to the tabIndices
arg.
The tabIndices
arg is a typescript Tuple of 2 number args. The first number will override the tabIndex of the currentElement
arg, while the second will override the nextElement
arg tabIndex. Note that both are optional based on our types, so you can provide one, the other, or both for granular override control. This allows for easy focus shifting/nav between 2 elements of any kind, including ones that should always stay interactable or not.
Implementation Examples ๐ญ
So how can this be used? Let's look at some unit tests I wrote for this function to see some examples of its potential:
const TestUI = () => {
return (
<div>
<button tabIndex={0}>Button 1</button>
<button tabIndex={-1}>Button 2</button>
</div>
);
};
it("should focus from one element to the next when provided a current and next element", async () => {
render(<TestUI />);
// focus the first button to start
await userEvent.keyboard("tab");
const button1 = screen.getByText("Button 1");
const button2 = screen.getByText("Button 2");
// focus from the first button to the second button using the util function
focusNewElement(button1, button2);
const focusedButton = document.activeElement;
expect(focusedButton).toHaveTextContent("Button 2");
});
it("should handle tabIndices by default from one element to the next when provided a current and next element", async () => {
render(<TestUI />);
// focus the first button to start
await userEvent.keyboard("tab");
const button1 = screen.getByText("Button 1");
const button2 = screen.getByText("Button 2");
// focus from the first button to the second button using the util function
focusNewElement(button1, button2);
expect(button1.tabIndex).toEqual(-1);
expect(button2.tabIndex).toEqual(0);
});
You can see from these test blocks how it works - call it with the current element and the element you want to shift to, it takes care of everything ๐. What used to be several lines of code per focus/keyboard nav shift now becomes one line.
How about those custom tabIndices? Well we tested that too - here's how you'd use it with those args:
it("should provide custom tabIndices to both elements if 2 numbers are passed in", async () => {
render(<TestUI />);
// focus the first button to start
await userEvent.keyboard("tab");
const button1 = screen.getByText("Button 1");
const button2 = screen.getByText("Button 2");
// focus from the first button to the second button using the util function
focusNewElement(button1, button2, [1, 2]);
expect(button1.tabIndex).toEqual(1);
expect(button2.tabIndex).toEqual(2);
});
As you can see, the tuple provides a super simple way to have full custom control over the tabIndex updates that happen programmatically, in just a few chars!
Other Considerations
It's worth noting as well our undefined
check for the tabIndices tuple values - someone will likely need to send in a 0 or -1 value of their own at some point, and without proper type checks those nums may evaluate as falsy, which would cause the override to not kick in. One of our tests validates that this behavior is prevented by our type checks:
it("should treat custom tabIndices as nums and not as truthy/falsy values", async () => {
render(<TestUI />);
// focus the first button to start
await userEvent.keyboard("tab");
const button1 = screen.getByText("Button 1");
const button2 = screen.getByText("Button 2");
// focus from the first button to the second button using the util function
focusNewElement(button1, button2, [0, -1]);
expect(button1.tabIndex).toEqual(0);
expect(button2.tabIndex).toEqual(-1);
});
๐ Conclusion
Nailing up-to-spec keyboard navigation in design system components is a great way to ensure high quality UX across products for assistive technology users. And outside of the design system, we know product teams will need similar utilities to ease the lift and repetition of keyboard nav code in UIs they're building.
By shipping this utility, we give ourselves and all frontend devs at Smartsheet a simple but powerful way to abstract out some of the verbose syntax that goes into keyboard nav/focusing. The result here is increased code readability, DRYness, quicker development time, and every so slightly less friction on our road to building accessible UIs for our users.
From here, bolstering our infrastructure for focus management and keyboard navigation across products is the goal. I think it'd be awesome to ship all kinds of focus utilities like this one, enabling consistency and DRY code across our own system and making the same possible for the consumers of our design system.
I hope this glimpse into our own UI a11y work inspires you to do similar stuff!
Cheers, Branon